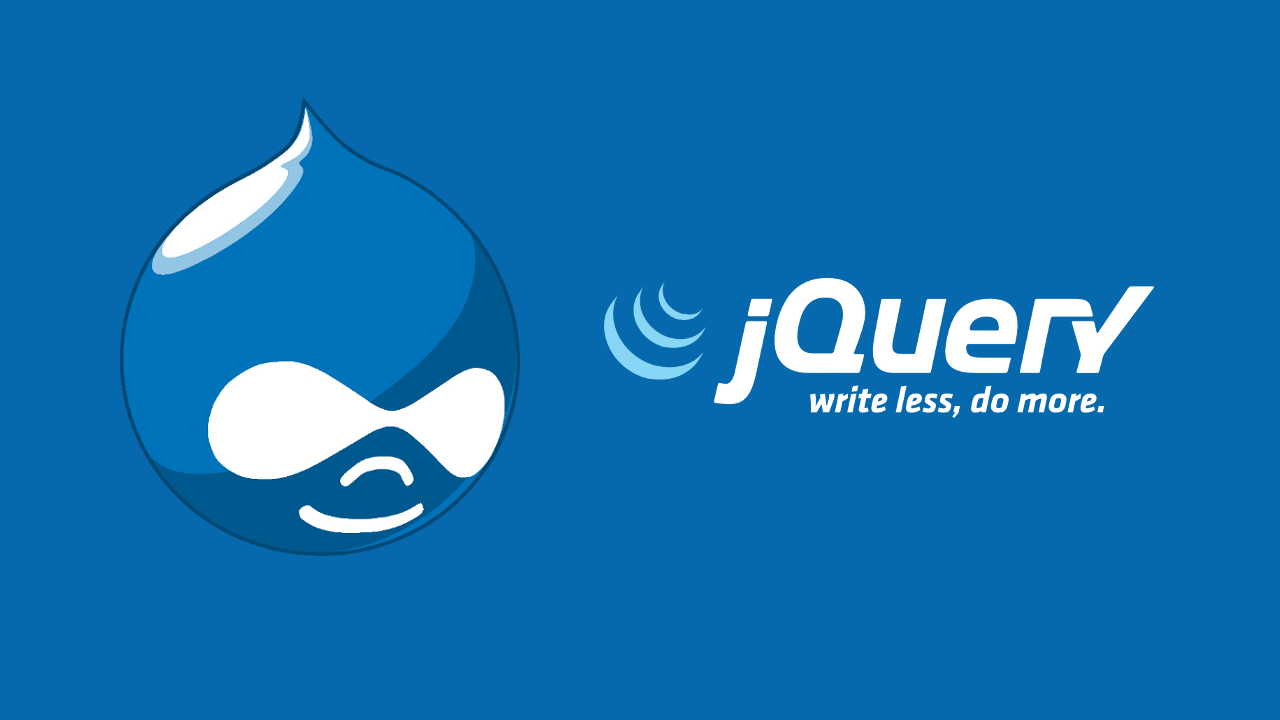
This article will present ten easy steps that will instantly improve your script’s performance. Don’t worry; there isn’t anything too difficult here. Everyone can apply these methods! When you’re finished reading, please let us know your speed tips.
1. Always Use the Latest Version
If you want to stay up to date without having to download the library a thousand times, GIYF (Google Is Your Friend), in this situation too. Google provides a lot ofAjax libraries from which to choose.
<!-- get the API with a simple script tag --> <script type="text/javascript" src="http://www.google.com/jsapi"></script> <script type="text/javascript"> /* and load minified jQuery v1.3.2 this way */ google.load ("jquery", "1.3.2", {uncompressed: false}); /* this is to display a message box when the page is loaded */ function onLoad () { alert ("jQuery + Google API!"); } google.setOnLoadCallback (onLoad); </script>
2. Combine and Minify Your Scripts
The majority of browsers are not able to process more than one script concurrently so they queue them up — and load times increase. Assuming the scripts are to be loaded on every page of your website, you should consider putting them all into a single file and use a compression tool (such as Dean Edwards’) to minify them. Smaller file sizes equal faster load times.
3. Use For Instead of Each
Native functions are always faster than any helper counterparts. Whenever you’re looping through an object received as JSON, you’d better rewrite your JSON and make it return an array through which you can loop easier.
Using Firebug, it’s possible to measure the time each of the two functions takes to run
var array = new Array (); for (var i=0; i<10000; i++) { array[i] = 0; } console.time('native'); var l = array.length; for (var i=0;i<l; i++) { array[i] = i; } console.timeEnd('native'); console.time('jquery'); $.each (array, function (i) { array[i] = i; }); console.timeEnd('jquery');
The above results are 2ms for native code, and 26ms for jQuery’s “each” method. Provided I tested it on my local machine and they’re not actually doing anything (just a mere array filling operation), jQuery’s each function takes over 10 times as long as JS native “for” loop. This will certainly increase when dealing with more complicated stuff, like setting CSS attributes or other DOM manipulation operations.
4. Use IDs Instead of Classes
It’s much better to select objects by ID because of the library’s behavior: jQuery uses the browser’s native method, getElementByID(), to retrieve the object, resulting in a very fast query.
So, instead of using the very handy class selection technique, it’s worth using a more complex selector (which jQuery certainly doesn’t fail to provide), write your own selector (yes, this is possible, if you don’t find what you need), or specify a container for the element you need to select
// Example creating a list and filling it with items // and selecting each item once console.time('class'); var list = $('#list'); var items = '<ul>'; for (i=0; i<1000; i++) { items += '<li class="item' + i + '">item</li>'; } items += '</ul>'; list.html (items); for (i=0; i<1000; i++) { var s = $('.item' + i); } console.timeEnd('class'); console.time('id'); var list = $('#list'); var items = '<ul>'; for (i=0; i<1000; i++) { items += '<li id="item' + i + '">item</li>'; } items += '</ul>'; list.html (items); for (i=0; i<1000; i++) { var s = $('#item' + i); } console.timeEnd('id');
The above code really shows the differences between the two ways of selecting elements, highlighting a never-ending over 5 seconds time to load the class driven snippet.
5. Give your Selectors a Context
The DOM node context originally passed to jQuery() (if none was passed then context will be equal to the document). It should be used in conjunction with the selector to determine the exact query used.
So, if you must use classes to target your elements, at least prevent jQuery from traversing the whole DOM using selectors appropriately.
Read More